Toggle buttons are useful for creating a group of buttons whose individual selection states all depend on a single variable. In this article, we’ll see the ways in which we can create and customize this component in Vuetify.
The v-btn-toggle Component
We can create a button group (or toggle button) in Vuetify with the v-btn-toggle
component. The group of buttons can be selected or toggled under a single v-model
, which is bound to the format
variable:
<template>
<v-app>
<v-row class="ma-2" justify="center">
<v-btn-toggle v-model="format" borderless>
<v-btn value="left">
<span>Left</span><v-icon right>mdi-format-align-left</v-icon>
</v-btn>
<v-btn value="center">
<span>Center</span><v-icon right>mdi-format-align-center</v-icon>
</v-btn>
<v-btn value="right">
<span>Right</span><v-icon right>mdi-format-align-right</v-icon>
</v-btn>
</v-btn-toggle>
</v-row>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
format: 'center',
}),
};
</script>

Clicking one of the buttons in the group unselects the currently selected one:

Ensuring a Toggle Button Value with the mandatory Prop
Vuetify provides the mandatory
prop, used to make sure that a toggle button will always have a value:
<template>
<v-app>
<v-row class="ma-2" justify="center">
<v-btn-toggle v-model="format" borderless mandatory>
<v-btn value="left">
<span>Left</span><v-icon right>mdi-format-align-left</v-icon>
</v-btn>
<v-btn value="center">
<span>Center</span><v-icon right>mdi-format-align-center</v-icon>
</v-btn>
<v-btn value="right">
<span>Right</span><v-icon right>mdi-format-align-right</v-icon>
</v-btn>
</v-btn-toggle>
</v-row>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
format: null,
}),
};
</script>

Vuetify Toggle Button Multiple Selection
The multiple
prop allows us to select more than one button in the group:
<template>
<v-app>
<v-row class="ma-2" justify="center">
<v-btn-toggle v-model="selectedLetters" multiple>
<v-btn v-for="(ch, index) in word" :key="index">{{ch}}</v-btn>
</v-btn-toggle>
</v-row>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
word: 'Beauty'.split(''),
selectedLetters: []
}),
};
</script>

Vuetify Rounded Toggle Buttons
We can make toggle buttons in Vuetify rounded with the rounded
prop:
<template>
<v-app>
<v-row class="ma-2" justify="center">
<v-btn-toggle v-model="letter" rounded>
<v-btn v-for="(ch, index) in word" :key="index">{{ ch }}</v-btn>
</v-btn-toggle>
</v-row>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
word: 'Coding'.split(''),
letter: '',
}),
};
</script>

Vuetify Borderless Toggle Buttons
Setting the borderless
prop to true
on a v-btn-toggle
will remove the borders from each button in the group.
<template>
<v-app>
<v-row class="ma-2" justify="center">
<v-btn-toggle v-model="letter" rounded borderless>
<v-btn v-for="(ch, index) in word" :key="index">{{ ch }}</v-btn>
</v-btn-toggle>
</v-row>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
word: 'Coding'.split(''),
letter: '',
}),
};
</script>

Customizing Toggle Button Colors in Vuetify
We can change the color of the selected button(s) in a button group with the color
prop:
<template>
<v-app>
<v-row class="ma-2" justify="center">
<v-btn-toggle v-model="selectedLetters" rounded multiple color="blue">
<v-btn v-for="(ch, index) in word" :key="index">{{ ch }}</v-btn>
</v-btn-toggle>
</v-row>
</v-app>
</template>
<script>
export default {
name: 'App',
data: () => ({
word: 'Beauty'.split(''),
selectedLetters: [],
}),
};
</script>

Summary
Toggle buttons are useful when we have a group of logically related buttons whose selection states need to be controlled by a single variable. Vuetify provides the v-btn-toggle component for creating them and various props for customization.
11 Amazing New JavaScript Features in ES13
This guide will bring you up to speed with all the latest features added in ECMAScript 13. These powerful new features will modernize your JavaScript with shorter and more expressive code.
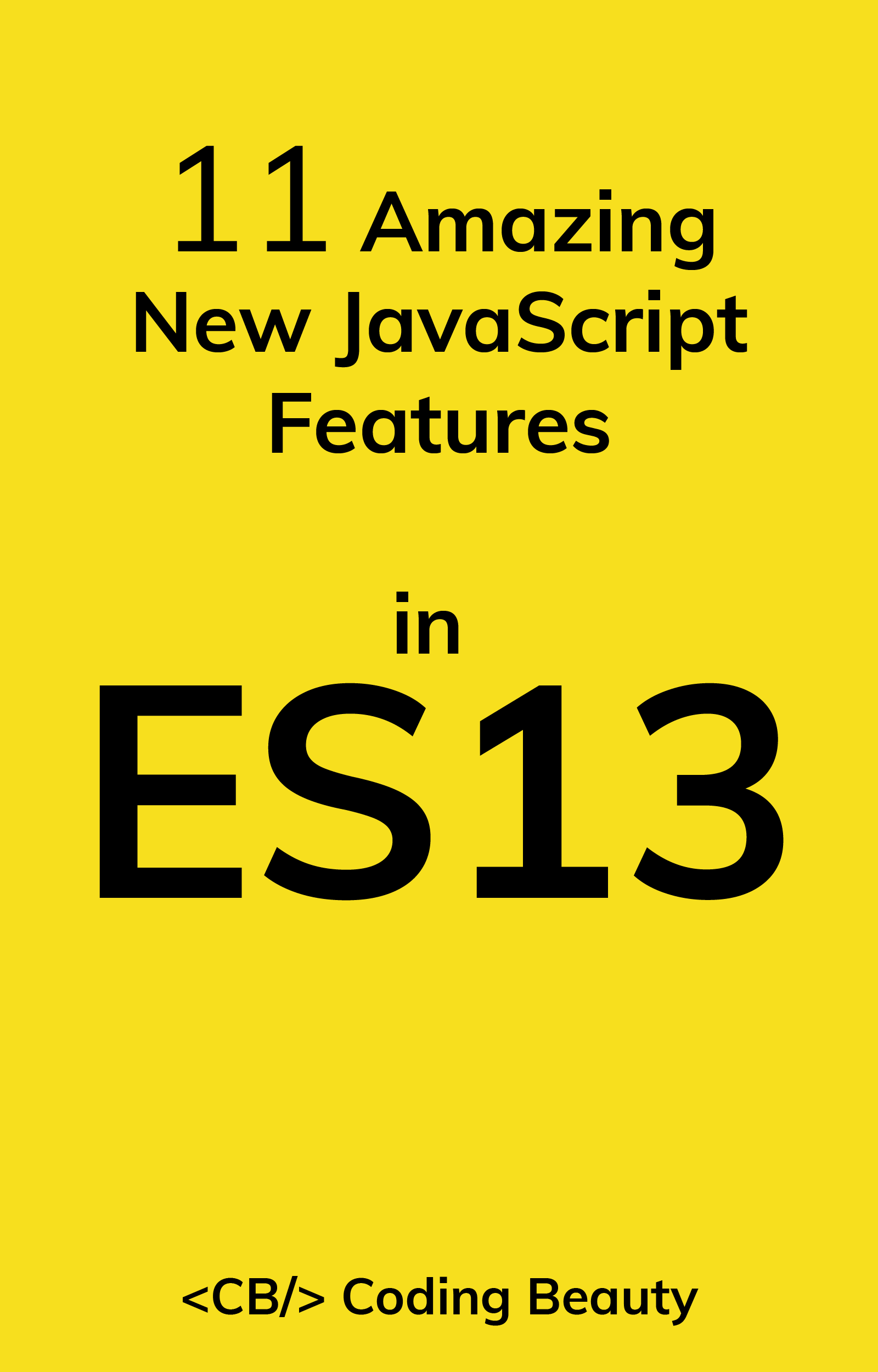